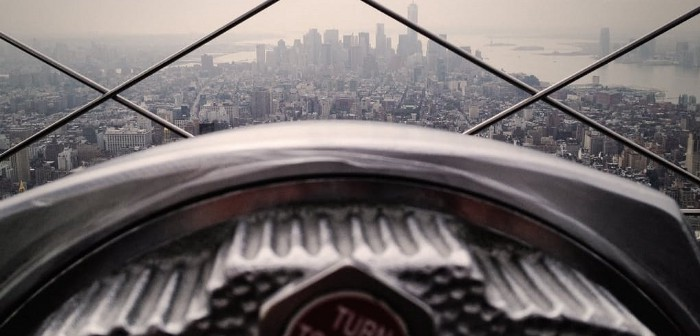
Vaadin 8 in Kotlin: Data model changes — ComboBox, Grid …
There are many nice features promised to come with Vaadin version 8. You probably know that Vaadin 8 is JDK 1.8 optimized version and many related simplifications are available in API now. Let’s look at some nice features related to data model closely. All examples below are created using Kotlin syntax.
ComboBox Items
Data source for ComboBox can be defined as a simple list of beans using setItems methods. Caption for ComboBox items displayed in a browser can be defined as generator using setItemCaptionGenerator method. It’s a real simplification as we can define a lambda for caption computation from bean properties. Kotlin language also brings some simplification by using ‘.apply’ method. We have less and more compact code.
val c = ComboBox<Person>().apply {
setItems(
listOf(
Person("John", "2"), Person("Matt", "1"),
Person("Jane", "3"), Person("Joe", "5")
)
)
setItemCaptionGenerator { it.name + " - " + it.id }
addValueChangeListener { println("Item selected ...") }
}
Grid — data providers
To set items for Grid component we can call setItems(listOf()) the same way we did for ComboBox above. But Grid component usually requires dynamic data, where we can add , remove or modify items and see Grid to refresh content by following our changes. For this purpose we can define DataProvider that loads our data from list. Now, whenever we update our gridData list, we can then call grid.dataProvider.refreshAll() to let Grid refresh items on screen. So simple and straightforward.
With Kotlin we used mutableListOf(…) to create list of beans quickly and ‘.apply’ to define Grid properties in one closed code block. The code looks quite nice and is easy to understand.
val gridData= mutableListOf(
Person("Mark", "2"), Person("John", "1"),
Person("Joe", "3"), Person("Dan", "4")
)
val grid = Grid<Person>().apply {
dataProvider = DataProvider.create(gridData)
addColumn { it.id }.setCaption("Id").setSortable(false)
addColumn { it.name }.setCaption("Name")
addSelectionListener {
Notification.show(it.firstSelected.get().name)
}
}