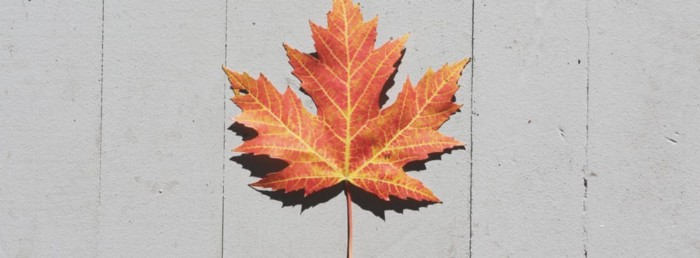
Spring Boot 1.4.x & Thymeleaf 3.x configuration
Sat, Mar 9, 2019
2-minute read
Thymeleaf is quite popular template engine widely used among Spring Boot developers. And we use it as well. While working with Spring Boot 1.4.x you will find that spring-boot-starter-thymeleaf is based on older Thymeleaf 2.x version, while there is Thymeleaf 3.x available for some time. But wait, there is an easy way how to get latest Thymeleaf for my Spring Boot 1.4.x application. There are 3 simple steps to do so.
- Reference Thymeleaf in build.gradle file (assuming we have Gradle based Spring Boot project)
compile("org.springframework.boot:spring-boot-starter-thymeleaf")
compile ("org.thymeleaf.extras:thymeleaf-extras-springsecurity4:3.0.0.RELEASE")
compile ("io.github.jpenren:thymeleaf-spring-data-dialect:3.1.1")
-
Specify Thymeleaf version in build.gradle file
Here is the place to say that we want version 3.x of Thymeleaf library
ext["thymeleaf.version"] = "3.0.2.RELEASE"
ext["thymeleaf-layout-dialect.version"] = "2.0.1"
- (Optional) Create
@Configuration
class with Thymeleaf configuration options. Spring Boot with detect this and create appropriate beans.
@Configuration
public class ThymeleafConfig extends WebMvcConfigurerAdapter implements ApplicationContextAware {
@Autowired
private MessageSource messageSource;
@Value("${thymeleaf.templates.cache}")
String thymeleafCache;
private ApplicationContext applicationContext;
public void setApplicationContext(ApplicationContext applicationContext) {
this.applicationContext = applicationContext;
}
@Bean
public ITemplateResolver templateResolver() {
SpringResourceTemplateResolver templateResolver = new SpringResourceTemplateResolver();
templateResolver.setApplicationContext(applicationContext);
templateResolver.setPrefix("classpath:/templates/");
templateResolver.setSuffix(".html");
templateResolver.setTemplateMode("HTML");
templateResolver.setCharacterEncoding("UTF-8");
if (thymeleafCache.equals("true")){
templateResolver.setCacheable(true);
} else {
templateResolver.setCacheable(false);
}
return templateResolver;
}
@Bean
public SpringTemplateEngine templateEngine() {
SpringTemplateEngine templateEngine = new SpringTemplateEngine();
templateEngine.setEnableSpringELCompiler(true);
templateEngine.setTemplateResolver(templateResolver());
templateEngine.setMessageSource(messageSource);
templateEngine.addDialect(new SpringDataDialect());
return templateEngine;
}
@Bean
public ViewResolver viewResolver() {
ThymeleafViewResolver viewResolver = new ThymeleafViewResolver();
viewResolver.setTemplateEngine(templateEngine());
return viewResolver;
}
}